As the title says, just an example for dynamic Menu and ToolBar-handling,
based on the Graphics-Classes (the Widget- and Form-Engine) of vbRichClient5.dll:
http://vbrichclient.com/#/en/Downloads.htm
The contained Modules of the Demo:
modMenuResources.bas
and modToolBarResources.bas
... contain the lines of code which are needed, to construct and achieve the following output:
MenuBar-DropDown:
Image may be NSFW.
Clik here to view.![]()
ToolBar-DropDown as the result of a DropArrow-Click (showing a dynamic PopUp-Menu):
Image may be NSFW.
Clik here to view.![]()
The constructed Menus use String-Keys to refer to previously loaded Icon and Image-Resources -
and they can be serialized to JSON-Strings (storable in a DB for example).
Any imaginable modern Alpha-Image-Resource can be used, as e.g. *.png, *.ico - but also
(as shown in the Demo) *.svg and *.svgz Vector-Images.
The example is completely ownerdrawn and truly independent from any MS-Menu-APIs, so one
can adapt *anything* as needed (e.g. the shape of the dropdown-form, colors, fonts, etc.) -
though the Demo as it is tries for a moderate style, mimicking a Win7-look roughly (with some
slight differences I personally like, but the whole thing is adaptable as said).
The code which implements this Menu-System is contained in one 'cf'-prefixed cWidgetForm-class
(cfPopUp for the hWnd-based Popups) - accompanied by 6 additional 'cw'-prefixed cWidgetBase-derived Classes:
cwMenuBar + cwMenuBarItem for the Menu-Strip
cwMenu + cwMenuItem for the DropDown-menus
cwToolBar + cwToolBarItem for the simple ToolBar-Implementation
I post this example more with regards to those, who want to learn how to program Widgets using
the vbRichClient-lib...
The above mentioned cwSomething Classes are programmable very similar to a VB-UserControl
(internally the same Event-Set is provided with KeyDown, MouseMove, MouseWheel, MouseEnter/MouseLeave etc.)
E.g. the cwToolBar-WidgetClass has only 100 lines of code - and the cwToolBarItem only 130 -
that's quite lean for what it does and offer, when you compare that with the efforts needed,
when "fighting" with SubClassing and SendMessage Calls against e.g. the MS-CommonControls. ;)
There's not a single Win-API-call throughout the implementation - but that's normal
for any framework, since they usually try to abstract from the underlying system.
The Menu- and ToolBar-Textrendering is Unicode-capable.
Have fun with it - here's the Zip-Download-Link: http://vbRichClient.com/Downloads/Me...oolbarDemo.zip
Olaf
based on the Graphics-Classes (the Widget- and Form-Engine) of vbRichClient5.dll:
http://vbrichclient.com/#/en/Downloads.htm
The contained Modules of the Demo:
modMenuResources.bas
Code:
Option Explicit
'this function returns a dynamically created Menu as a JSON-String (which could be stored in a DB, or elsewhere)
Public Function ExampleMenuAsJSONString() As String
Dim Root As cMenuItem
Set Root = Cairo.CreateMenuItemRoot("MenuBar", "MenuBar")
AddFileMenuEntriesTo Root.AddSubItem("File", "&File")
AddEditMenuEntriesTo Root.AddSubItem("Edit", "&Edit")
AddEditMenuEntriesTo Root.AddSubItem("Disabled", "&Disabled", , False) 'just to demonstrate a disabled entry
AddExtrMenuEntriesTo Root.AddSubItem("Extras", "E&xtras")
AddHelpMenuEntriesTo Root.AddSubItem("Help", "&Help")
ExampleMenuAsJSONString = Root.ToJSONString
End Function
Public Sub AddFileMenuEntriesTo(MI As cMenuItem)
MI.AddSubItem "New", "&New", "Document-New"
MI.AddSubItem "Sep", "-"
MI.AddSubItem "Open", "&Open...", "Document-Open"
MI.AddSubItem "Save", "&Save", "Document-Save"
MI.AddSubItem "SaveAs", "&Save as...", "Document-Save-As"
MI.AddSubItem "Sep2", "-"
MI.AddSubItem "ExitApp", "E&xit Application", "Application-Exit"
End Sub
Public Sub AddEditMenuEntriesTo(MI As cMenuItem)
MI.AddSubItem "Cut", "C&ut", "Edit-Cut"
MI.AddSubItem "Copy", "&Copy", "Edit-Copy"
MI.AddSubItem "Paste", "&Paste", "Edit-Paste", CBool(Len(New_c.Clipboard.GetText))
MI.AddSubItem "Delete", "&Delete", "Edit-Delete"
MI.AddSubItem "Sep", "-" '<- a Menu-Separatorline-Definiton
MI.AddSubItem "Select all", "&Select all", "Edit-Select-All"
End Sub
Public Sub AddExtrMenuEntriesTo(MI As cMenuItem)
Dim SubMenuPar As cMenuItem, SubSubMenuPar As cMenuItem
MI.AddSubItem "Item1", "Menu-Item&1", "MenuIconKey1"
MI.AddSubItem "Item2", "Menu-Item&2", "MenuIconKey3", False
MI.AddSubItem "Item3", "-" '<- a Menu-Separatorline-Definiton
MI.AddSubItem "Item4", "&Menu-Item2 disabled", "MenuIconKey1", , True
Set SubMenuPar = MI.AddSubItem("Item5", "This pops up a &SubMenu", "MenuIconKey2")
'two entries into the SubMenu (as children of 'Item5' of the above Code-Block)
SubMenuPar.AddSubItem "SubItem1", "Caption SubItem1", "MenuIconKey1"
Set SubSubMenuPar = SubMenuPar.AddSubItem("SubItem2", "Caption SubItem2", "MenuIconKey2")
'and just 1 entry into the SubSubMenu (children of 'SubItem2' of the above Code-Block)
SubSubMenuPar.AddSubItem "SubSubItem1", "Caption SubSubItem1", "MenuIconKey1"
End Sub
Public Sub AddHelpMenuEntriesTo(MI As cMenuItem)
MI.AddSubItem "About", "&About", "About-Hint"
MI.AddSubItem "Sep", "-"
MI.AddSubItem "Index", "&Index...", "Help-Contents"
MI.AddSubItem "Find", "&Find...", "Edit-Find"
End Sub
Code:
Option Explicit
Public Sub CreateToolBarEntriesOn(ToolBar As cwToolBar)
ToolBar.AddItem "Home", "go-home", , , "normal Icon with 'IsCheckable = True'", , True
ToolBar.AddItem "Undo", "go-previous", , , "normal Icon"
ToolBar.AddItem "Redo", "go-next", , , "disabled Icon", False
ToolBar.AddItem "Search", "page-zoom", , ddDropDown, "Icon with DropDownArrow"
ToolBar.AddItem "Sep", , "-", , "Separator-Line"
ToolBar.AddItem "TxtItem1", , "TxtItem1", , "plain Text-Item"
ToolBar.AddItem "TxtItem2", "Document-Save-As", "TxtItem2", , "Text-Item with Icon"
ToolBar.AddItem "Sep2", , "-", , "Separator-Line"
ToolBar.AddItem "TxtItem3", , "TxtItem3", ddDropDown, "Text-Item with DropDown"
ToolBar.AddItem "TxtItem4", "Edit-Find", "TxtItem4", ddDropDown, "Text-Item with Icon and DropDown"
ToolBar.AddItem "Sep3", , "-", , "Separator-Line"
ToolBar.AddItem "TxtItem5", "Document-Open", "TxtItem5", ddCrumbBar, "Text-Item with Icon and CrumbBar-Style-DropDown"
ToolBar.AddItem "TxtItem6", , "TxtItem6", ddCrumbBar, "Text-Item with CrumbBar-Style-DropDown"
ToolBar.AddItem "TxtItem7", , "TxtItem7", , "plain Text-Item"
End Sub
MenuBar-DropDown:
Image may be NSFW.
Clik here to view.
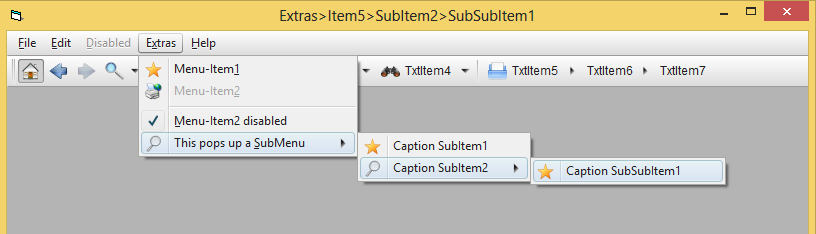
ToolBar-DropDown as the result of a DropArrow-Click (showing a dynamic PopUp-Menu):
Image may be NSFW.
Clik here to view.
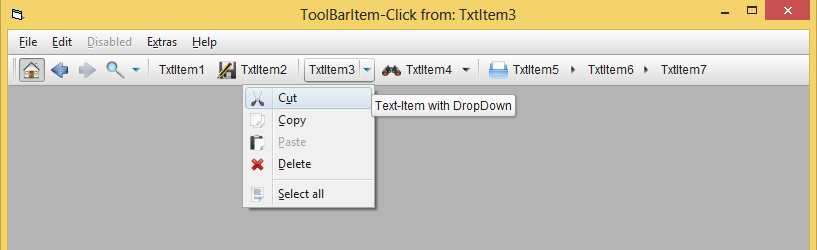
The constructed Menus use String-Keys to refer to previously loaded Icon and Image-Resources -
and they can be serialized to JSON-Strings (storable in a DB for example).
Any imaginable modern Alpha-Image-Resource can be used, as e.g. *.png, *.ico - but also
(as shown in the Demo) *.svg and *.svgz Vector-Images.
The example is completely ownerdrawn and truly independent from any MS-Menu-APIs, so one
can adapt *anything* as needed (e.g. the shape of the dropdown-form, colors, fonts, etc.) -
though the Demo as it is tries for a moderate style, mimicking a Win7-look roughly (with some
slight differences I personally like, but the whole thing is adaptable as said).
The code which implements this Menu-System is contained in one 'cf'-prefixed cWidgetForm-class
(cfPopUp for the hWnd-based Popups) - accompanied by 6 additional 'cw'-prefixed cWidgetBase-derived Classes:
cwMenuBar + cwMenuBarItem for the Menu-Strip
cwMenu + cwMenuItem for the DropDown-menus
cwToolBar + cwToolBarItem for the simple ToolBar-Implementation
I post this example more with regards to those, who want to learn how to program Widgets using
the vbRichClient-lib...
The above mentioned cwSomething Classes are programmable very similar to a VB-UserControl
(internally the same Event-Set is provided with KeyDown, MouseMove, MouseWheel, MouseEnter/MouseLeave etc.)
E.g. the cwToolBar-WidgetClass has only 100 lines of code - and the cwToolBarItem only 130 -
that's quite lean for what it does and offer, when you compare that with the efforts needed,
when "fighting" with SubClassing and SendMessage Calls against e.g. the MS-CommonControls. ;)
There's not a single Win-API-call throughout the implementation - but that's normal
for any framework, since they usually try to abstract from the underlying system.
The Menu- and ToolBar-Textrendering is Unicode-capable.
Have fun with it - here's the Zip-Download-Link: http://vbRichClient.com/Downloads/Me...oolbarDemo.zip
Olaf